Single Sign-On Made Easy: A Guide to Implementing SSO in Node.js
In this blog post, I explain Single Sign-On (SSO) and its benefits for businesses. I cover the prerequisites for implementing SSO and dive into how it works with Node.js. Additionally, I discuss the most popular SSO protocols and provide real-world examples of SSO in action.
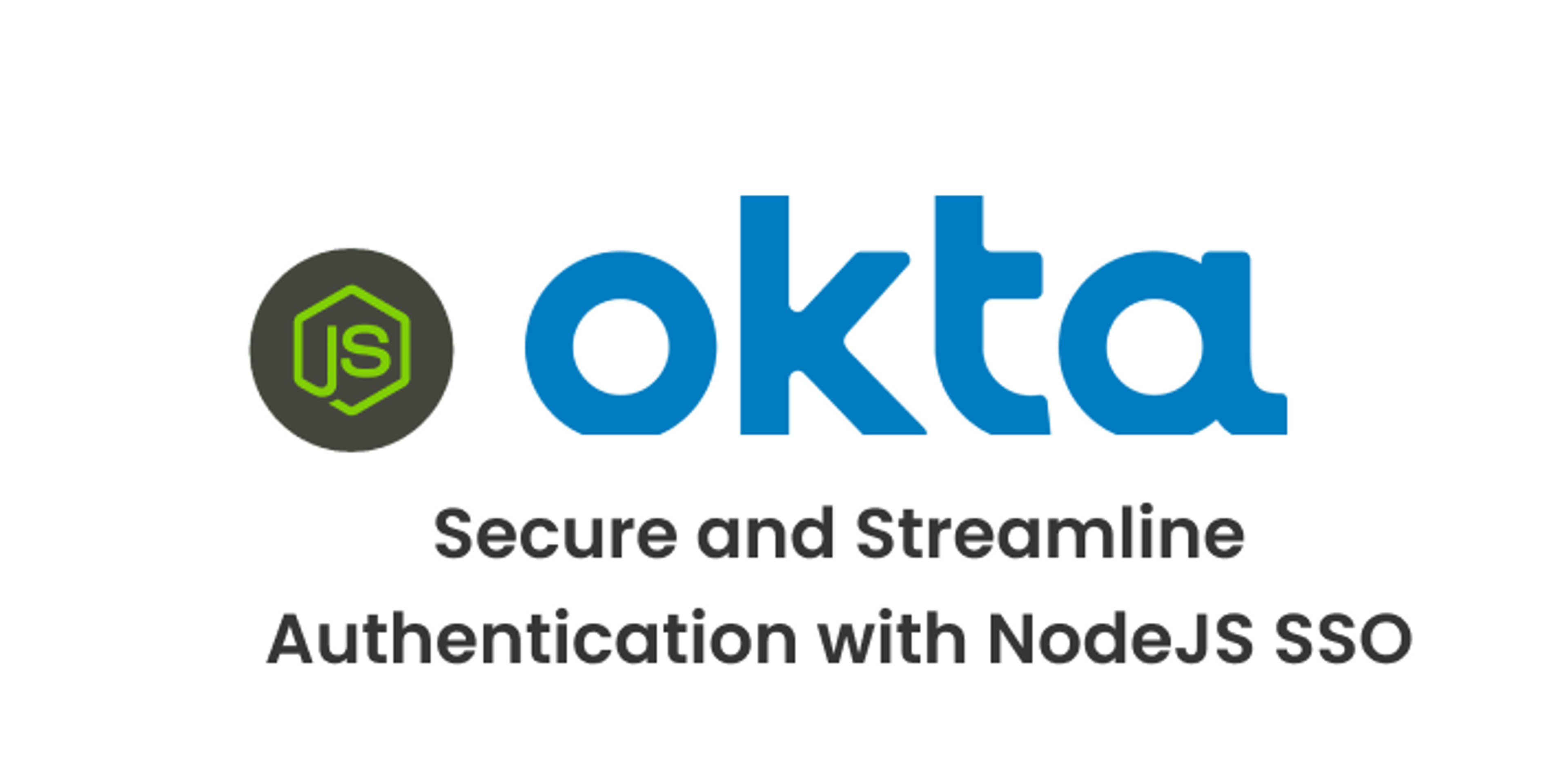
Single Sign-On (SSO) is an authentication process that enables users to access multiple applications with a single set of login credentials. SSO eliminates the need for users to remember different login credentials for each application, which makes the login process more convenient and efficient.
Node.js is a popular JavaScript runtime environment that can be used to implement SSO functionality. In this blog post, we'll explore how to implement SSO in Node.js and discuss its pros and cons.
Prerequisites:
- Basic knowledge of Node.js
- Understanding of web authentication protocols such as OAuth2 and OpenID Connect
Implementing SSO in Node.js
To implement SSO in Node.js, we can use an SSO provider such as Okta, Auth0, or AWS Cognito. These providers offer easy-to-use APIs that can be integrated into Node.js applications.
Here's an overview of how SSO works with Node.js:
- The user logs in to the SSO provider using their credentials.
- The SSO provider generates an access token and returns it to the user.
- The user sends the access token to the Node.js application.
- The Node.js application verifies the access token with the SSO provider and grants access to the user.
To implement this flow in Node.js, we can use popular libraries such as Passport.js and Node OIDC Provider. Passport.js provides a middleware-based authentication framework that can be used with different authentication providers, including SSO providers. Node OIDC Provider is a library that enables us to create our own OpenID Connect Provider (OP) in Node.js.
Here's an example of using Passport.js with an SSO provider:
1const passport = require('passport');
2const { Strategy: OpenIDStrategy } = require('passport-openidconnect');
3
4passport.use(new OpenIDStrategy({
5 issuer: 'https://example.okta.com/oauth2/default',
6 clientID: 'YOUR_CLIENT_ID',
7 clientSecret: 'YOUR_CLIENT_SECRET',
8 callbackURL: 'http://localhost:3000/auth/callback',
9 scope: 'openid profile email'
10}, (issuer, sub, profile, accessToken, refreshToken, done) => {
11 // Authentication logic here
12 done(null, profile);
13}));
14
15app.get('/auth/login', passport.authenticate('openidconnect'));
16
17app.get('/auth/callback', passport.authenticate('openidconnect', {
18 failureRedirect: '/login'
19}), (req, res) => {
20 // Redirect to success page
21 res.redirect('/success');
22});
This example uses the passport-openidconnect
library to implement SSO with Okta as the authentication provider.
Pros and Cons of SSO in Node.js
Pros
- Simplifies the login process for users, increasing convenience and efficiency.
- Reduces the risk of password fatigue and increases security by enabling users to use a single set of credentials across multiple applications.
- Provides a centralized authentication mechanism, which can be easier to manage and maintain.
Cons
- Reliance on a third-party authentication provider may introduce a single point of failure.
- Integration with existing systems can be complex and require significant effort.
- Users may be required to provide additional personal information to the authentication provider.
Use Cases
SSO can be beneficial in a wide range of scenarios, including:
- Large organizations with multiple internal applications.
- SaaS companies with multiple applications and services.
- Educational institutions with multiple web-based resources.
- Websites with a large user base that want to simplify the login process.
Conclusion
Implementing SSO in Node.js can simplify the login process for users and reduce the risk of password fatigue. By using popular libraries such as Passport.js and Node OIDC Provider
🔖 About the Author

Ankur Datta
dev.ankur.datta@gmail.com
Ankur Datta: self-proclaimed cool dude and tech wiz. He can code circles around most mortals and talk tech jargon with the best of them.